Using JWT to Invoke External Service in Salesforce
JSON Web Token, or JWT, is a way to enable authentication and get you access to a resource. It is a digitally signed token that can be used for authentication across parties, and is a self-contained way to transfer information and include claims to specified resources. It is common to use JWT in stateless web applications to provide a easy, seamless way to authenticate requests.
We can use JWT in Salesforce Apex quite easily. We will use a sample service to demonstrate the code.
Simple Way to use JWT #
Create a new class TestApiJwt
.
Input below code -
public with sharing class TestApiJwt {
public static void getUsersSimple(){
String jwtToken = '';
HttpRequest req = new HttpRequest();
req.setEndpoint('https://reqres.in/api/users');
req.setMethod('GET');
req.setHeader('Authorization', 'Bearer ' + jwtToken);
Http http = new Http();
HttpResponse res = http.send(req);
Map<String, Object> response;
if (res.getStatusCode() == 200 ) {
response = (Map<String, Object>)JSON.deserializeUntyped(res.getBody());
System.debug('response: ' + response);
}
else {
System.debug('Error');
}
}
}
We do a few things here -
- Create an object of type
HttpRequest
calledreq
- Set end pont to a sample URL (
https://reqres.in/api/users
) - Set JWT in request header
- Invoke
GET
method withHttpResponse res = http.send(req)
- Check response object for status code
200
that denotes a successful request -res.getStatusCode()
- Print out the response
(Remember to deploy the code to your org if you are working in VSCode!)
Now, let us call this class in an anonymous execution window. In VSCode, create a new file RunClass
in scripts/apex/
-
TestApiJwt.getUsersSimple();
Run the code to see output..
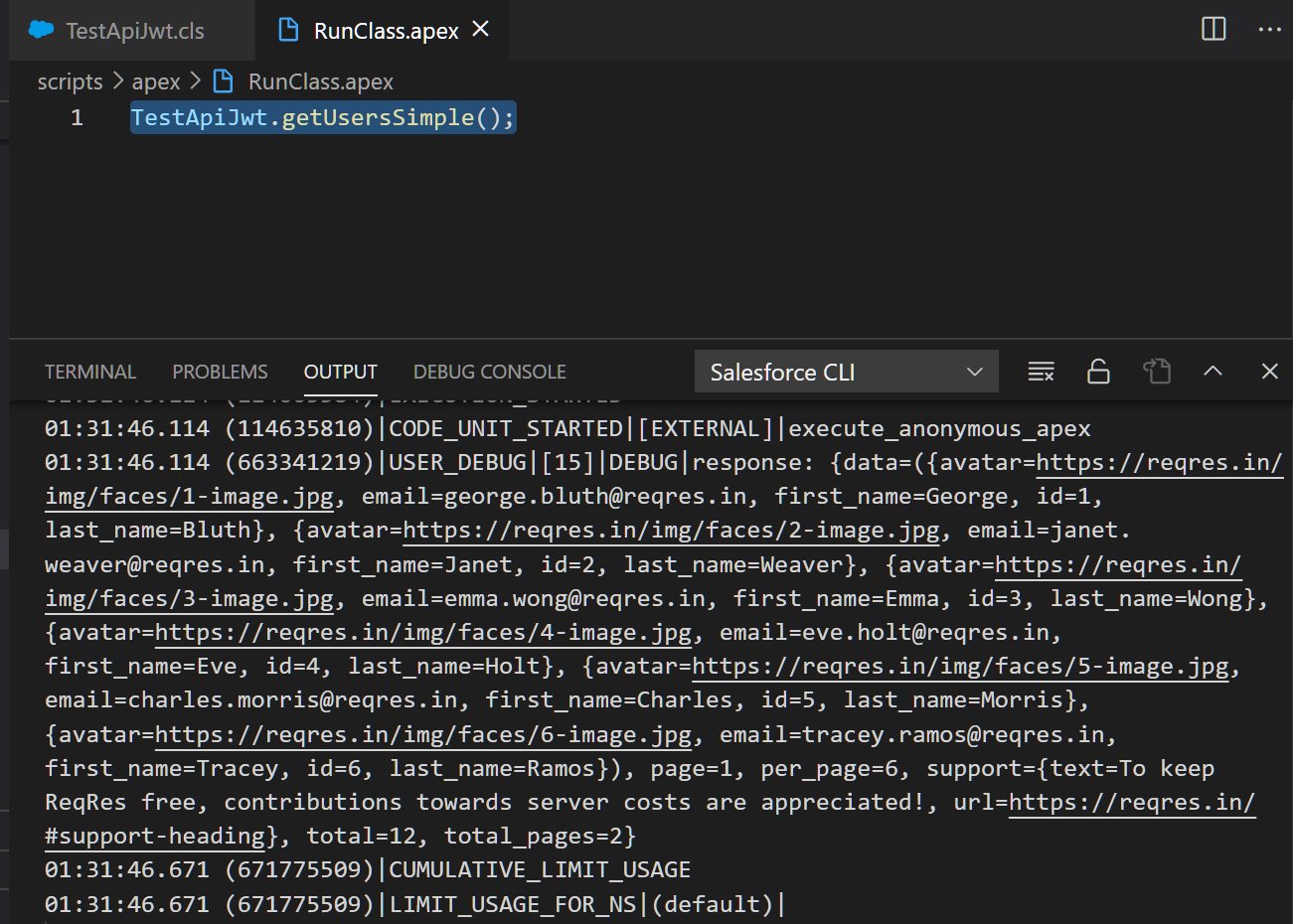
This method is crude and may not be used in secure environments!
A More Sophisticated Way to use JWT #
Use named credentials.
First, create a self-signed or CA-signed certificate. Go to Setup
> Certificate and Key Management
. Click Create Self-Signed Certificate
and create a certificate if none exists for the purpose.
Go to Setup
> Security
> Named Credentials
. Create a new named credential -
Label: TestJwt
URL: https://reqres.in/api/
Certificate: <select certificate you created>
Identity Type: Named Principal
Authentication Protocol: JWT
Issuer: Self
Named Principal Subject: NA
Named Principal Subject: NA
Token Valid For: 9000 seconds
Next, setup your external service to recognize this JWT. Note that this step may vary depending on whether you choose JWT
or JWT Exchange
as Authentication Protocol
.
JWT
option will need your external service to recognize Salesforce created tokenJWT Exchange
allows you to trigger an auth flow, get the valid JWT generated by external service, and use that token for the transaction.
All you do in your code is to invoke URL through this named credential.
HttpRequest req = new HttpRequest();
req.setEndpoint('callout:TestJwt/users');
req.setMethod('GET');
Http http = new Http();
HTTPResponse res = http.send(req);
System.debug(res.getBody());
We used similar code as last time, but called out through our named credential instead of hard-coding the JWT.
Final Words #
While JWT is useful for establishing trust across servers/applications, it’s not quite popular for point-to-point communication, or in situations where different application servers need more control over the authentication/authorization mechanism.